Software Design
This article is mainly a list of conceptual resources about building a web application.
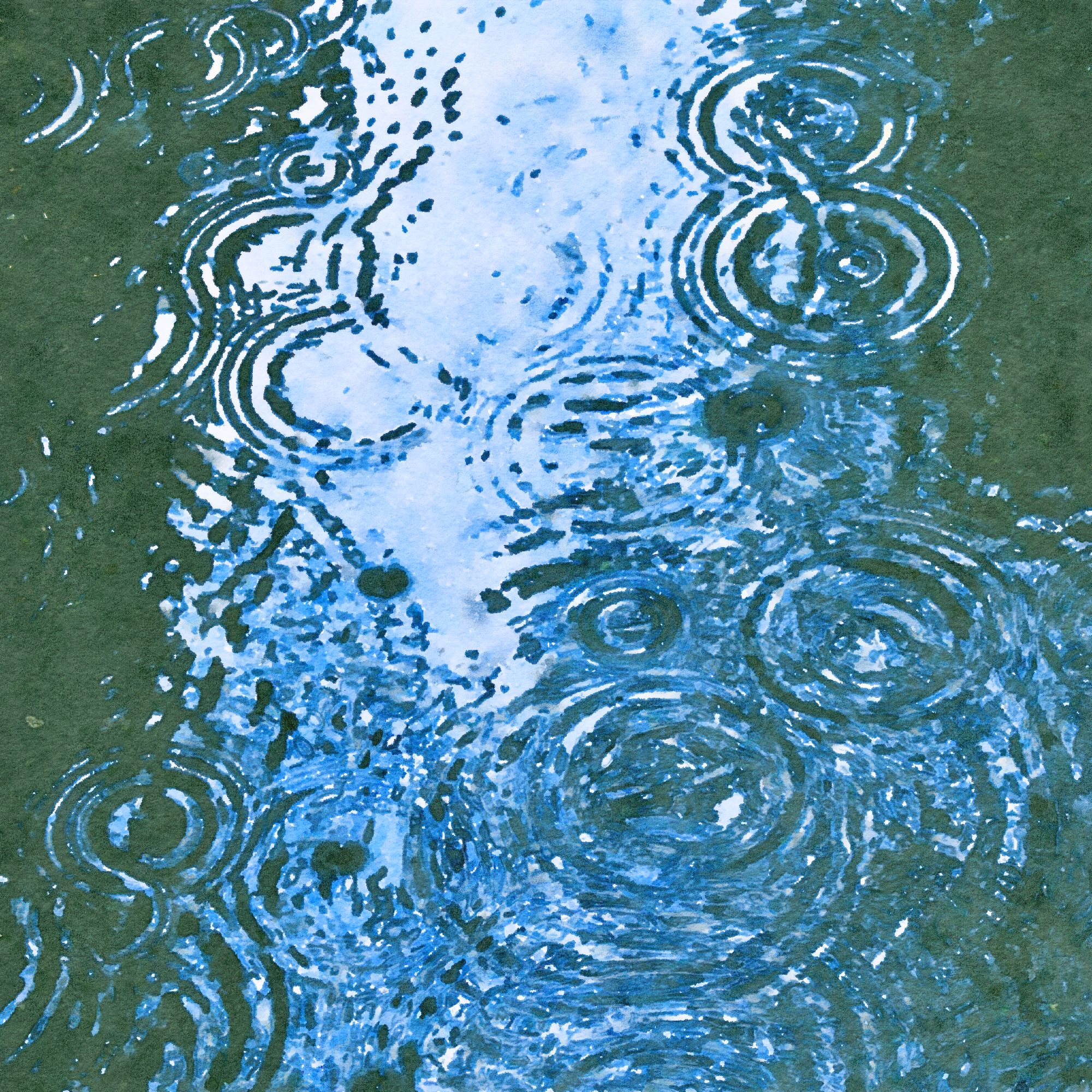
"Software design usually involves problem-solving and planning a software solution. This includes both a low-level component and algorithm design and a high-level, architecture design."
https://en.wikipedia.org/wiki/Software_design
In the last article, we started working with NPM, which bundles with Node.js. Node is just one component of a software solution.
We may forget to ask questions as a result of following generally accepted solutions. What are the system components, and why use them? How do parts of the system interact? So let's take a few minutes to understand building a web application with the MERN stack.
As an overview, I do not include a look at alternative solutions, pros and cons, security, requirements, testing, performance, reliability, deployment, cost, and so forth. Detail about the architecture of the Internet and Web may be relevant here but almost entirely avoided for the sake of simplicity.
By seeing the big-picture, we can better understand the context for what we're building.
First, what is a web application?
"A web application (or web app) is application software that runs on a web server, unlike computer-based software programs that are run locally on the operating system (OS) of the device. Web applications are accessed by the user through a web browser with an active network connection."
https://en.wikipedia.org/wiki/Web_application
Second, why build for the web instead of a native application?
Any device with a modern browser and Internet connection can access your app. In addition, software updates deliver on reload, and no store review process or developer fees are required. However, these points are an oversimplification. Also, it's not uncommon to develop on multiple platforms.
https://en.wikipedia.org/wiki/Mobile_app
Software architecture
"Software architecture refers to the fundamental structures of a software system and the discipline of creating such structures and systems."
https://en.wikipedia.org/wiki/Software_architecture
Stack
"In computing, a solution stack or software stack is a set of software subsystems or components needed to create a complete platform such that no additional software is needed to support applications."
https://en.wikipedia.org/wiki/Solution_stack
Front end and back end
"In software engineering, the terms front end and back end refer to the separation of concerns between the presentation layer (front end), and the data access layer (back end) of a piece of software, or the physical infrastructure or hardware."
https://en.wikipedia.org/wiki/Front_end_and_back_end
Full-stack
"In technology development, full stack refers to an entire computer system or application from the front end to the back end and the code that connects the two."
https://www.webopedia.com/definitions/full-stack/
MERN
The MERN stack is MongoDB, Express, React, and Node. You build the front-end with React, which leverages JavaScript, HTML, and CSS. Node, Express, and MongoDB form the back-end—also constructed with JavaScript. Thus, we can use JavaScript across the entire stack with this solution instead of using different programming languages for the front-end and back-end.
MongoDB
"MongoDB is a source-available cross-platform document-oriented database program. Classified as a NoSQL database program, MongoDB uses JSON-like documents with optional schemas." The development of MongoDB is mainly with C++ and Javascript; it uses a server-side public license. The initial release was in 2009.
https://en.wikipedia.org/wiki/MongoDB
Express.js
"Express.js, or simply Express, is a back end web application framework for Node.js, released as free and open-source software under the MIT License. It is designed for building web applications and APIs. It has been called the de facto standard server framework for Node.js." Express uses Javascript as its development language, and the initial release was in 2010.
https://en.wikipedia.org/wiki/Express.js
React.js
"React (also known as React.js or ReactJS) is a free and open-source front-end JavaScript library for building user interfaces or UI components." React is written in Javascript and is open-source with an MIT license; the initial release was in 2013.
https://en.wikipedia.org/wiki/React_(JavaScript_library)
Node.js
"Node.js is an open-source, cross-platform, back-end JavaScript runtime environment that runs on the V8 engine and executes JavaScript code outside a web browser." Node mainly uses Javascript, C++, and Python; it has an open-source MIT license, and the initial release was in 2009.
https://en.wikipedia.org/wiki/Node.js
Open-source software
"Open-source software (OSS) is computer software that is released under a license in which the copyright holder grants users the rights to use, study, change, and distribute the software and its source code to anyone and for any purpose."
https://en.wikipedia.org/wiki/Open-source_software
https://opensource.com/resources/what-open-source
Client-server model
"Client–server model is a distributed application structure that partitions tasks or workloads between the providers of a resource or service, called servers, and service requesters, called clients."
https://en.wikipedia.org/wiki/Client–server_model
Web browser
"When a user requests a web page from a particular website, the web browser retrieves the necessary content from a web server and then displays the page on the user's device."
https://en.wikipedia.org/wiki/Web_browser
Uniform Resource Identifier
"A URL provides the location of the resource. A URI identifies the resource by name at the specified location or URL."
"As such, a URL is simply a URI that happens to point to a resource over a network."
https://en.wikipedia.org/wiki/Uniform_Resource_Identifier
Representational state transfer
"In a RESTful Web service, requests made to a resource's URI elicit a response with a payload formatted in HTML, XML, JSON, or some other format."
https://en.wikipedia.org/wiki/Representational_state_transfer
Request methods
"HTTP defines methods… to indicate the desired action to be performed on the identified resource."
https://en.wikipedia.org/wiki/Hypertext_Transfer_Protocol#Request_methods
Web API
"A Web API is an application programming interface for either a web server or a web browser."
"A server-side web API is a programmatic interface consisting of one or more publicly exposed endpoints to a defined request-response message system, typically expressed in JSON or XML, which is exposed via the web—most commonly by means of an HTTP-based web server."
https://en.wikipedia.org/wiki/Web_API
https://www.programmableweb.com/api-university/what-are-apis-and-how-do-they-work
Dynamic web page
"A server-side dynamic web page is a web page whose construction is controlled by an application server processing server-side scripts."
https://en.wikipedia.org/wiki/Dynamic_web_page
"With Ajax, web applications can send and retrieve data from a server asynchronously (in the background) without interfering with the display and behavior of the existing page."
"The webpage can be modified by JavaScript to dynamically display—and allow the user to interact with the new information. The built-in XMLHttpRequest object is used to execute Ajax on webpages, allowing websites to load content onto the screen without refreshing the page."
https://en.wikipedia.org/wiki/Ajax_(programming)
Single-page application
"A single-page application (SPA) is a web application or website that interacts with the user by dynamically rewriting the current web page with new data from the web server, instead of the default method of a web browser loading entire new pages. The goal is faster transitions that make the website feel more like a native app."
https://en.wikipedia.org/wiki/Single-page_application
Responsive web design
"Responsive web design (RWD) is an approach to web design that makes web pages render well on a variety of devices and window or screen sizes from minimum to maximum display size."
https://en.wikipedia.org/wiki/Responsive_web_design
Progressive web application
"A progressive web application (PWA) is a type of application software delivered through the web, built using common web technologies including HTML, CSS and JavaScript. It is intended to work on any platform that uses a standards-compliant browser, including both desktop and mobile devices."
https://en.wikipedia.org/wiki/Progressive_web_application
https://web.dev/what-are-pwas/
Summary
Full-stack web applications run in the browser, but behave like native apps. The user interacts through a front-end interface, which makes requests to a server back-end for data. Resource requests and responses occur through a web API with defined URI paths. Parts of the webpage can be constructed or modified at various times without reloading. Different open-source web frameworks help "provide a standard way to build and deploy web applications on the World Wide Web."
Next up is learning about NPM packages and configuring files that Git should ignore.