Working with GitHub
Start working with GitHub using the command line.
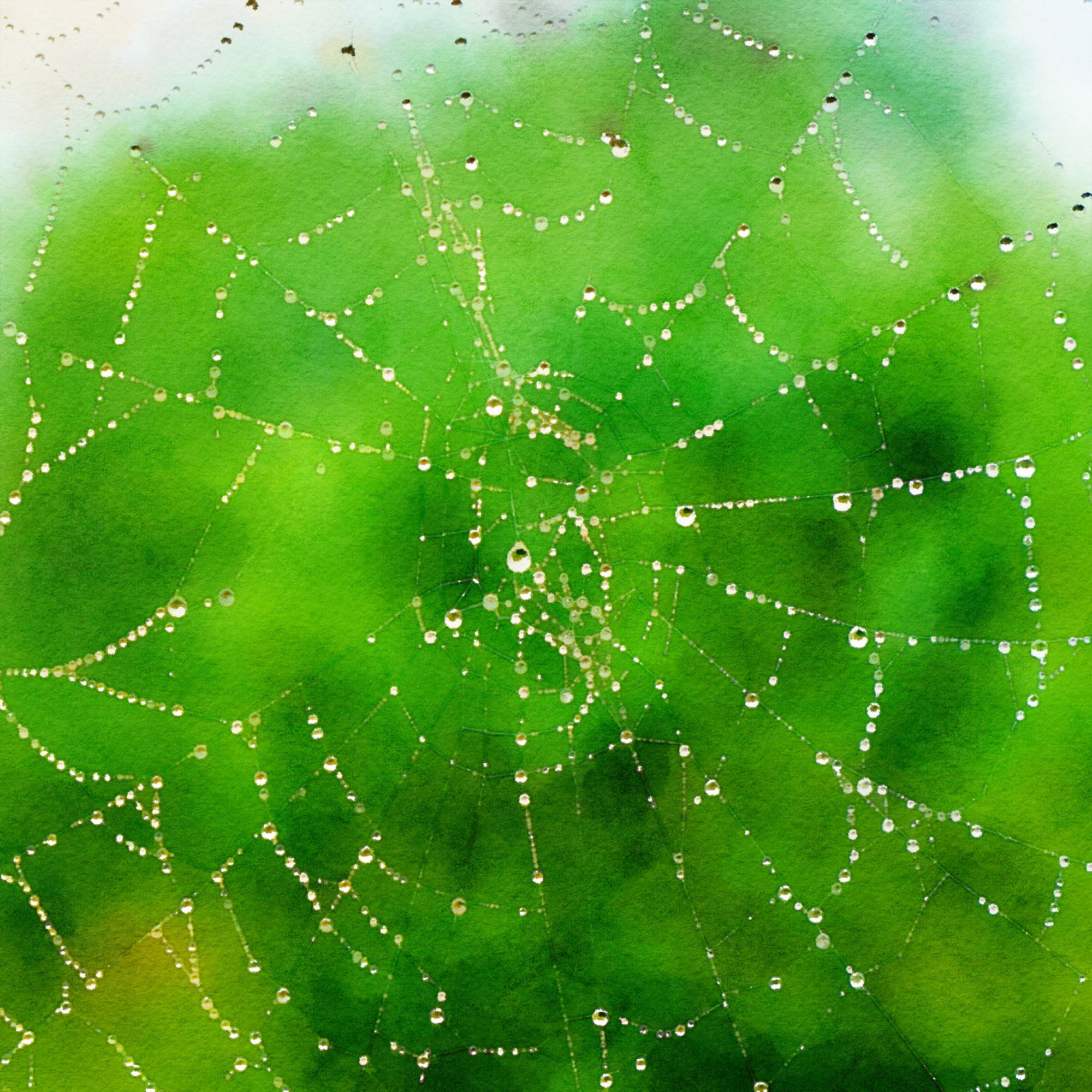
In the previous article, we set up a server with Express. For this article, we'll start working with GitHub using the command line. The main reason to use a service like GitHub is to collaborate with other people on a project. GitHub offers tools to share and manage code from different developers across the Internet. The setup step requires you to sign-up for an account. You'll set up secure communication from your computer to GitHub.
There are two main workflows for new projects on GitHub. The first is to create a project on GitHub and clone the project to your local machine. The second is to start with a local project and upload it to an empty existing project on GitHub. Generally, the first option is the easiest, but sometimes you develop a project locally and upload it to GitHub later.
GitHub account setup
Creating a new GitHub account is relatively straightforward. The GitHub docs are a great place to go if you have questions.
https://docs.github.com/en/account-and-profile
In a previous tutorial, we set up a default Git email. You can revisit the following GitHub documentation for further guidance.
Creating a new project
Projects on GitHub are called repositories or repos for short. Repos give you a place to store your code and manage how you want to share it with other people.
https://docs.github.com/en/repositories
You generally default to your profile page after creating an account and signing in. However, if needed, you can navigate to your profile by visiting https://github.com, then click on the user icon in the upper right-hand corner of the window.
There are various ways to create a new project. First, you can click on the plus icon in the upper right-hand corner and select "New Repository." Next, you'll see the "Create a new repository" page.
Alternately, you can click on the user icon (as before) and select "Your repositories" from the drop-down list. Then, toward the upper right-hand corner of the window, there is a green button called "New." After clicking the New button, you'll see the "Create a new repository" page (as above).
https://docs.github.com/en/repositories/creating-and-managing-repositories/creating-a-new-repository
Give your repository a name, select private or public, and mark the checkbox to add a read me file. In a previous tutorial, we learned about git ignore files.
Click the checkbox to add a git ignore file and search for Node in the "filter ignores…".
Also, choose a license and select whatever fits your needs best. "Learn more" is a helpful link that provides valuable resources for licensing. Finally, click the green "Create repository" button.
This page lists GitHub license keywords that you can later add to your package.json file.
You now have a new project on GitHub! The next step is to download that code to your computer.
Cloning a GitHub repository
There is a green "Code" button on the new repository page. Click the Code button and notice three cloning options: HTTPS, SSH, and GitHub CLI. First, copy the repo URL (click the copy icon).
Next, open up the Terminal to access the command line. Finally, navigate to the Desktop or wherever you want the project to be stored.
cd Desktop
To download the project type, git clone
(space) and then paste (command + v) the URL from GitHub, so it looks like this.
git clone https://github.com/yourusername/yourrepository.git
Hit enter
to execute the command. Next, you'll see a prompt for your GitHub username and password.
Cloning into 'yourrepository'...
Username for 'https://github.com':
Password for …
Result:
remote: Support for password authentication was removed on August 13, 2021. Please use a personal access token instead.
remote: Please see https://github.blog/2020-12-15-token-authentication-requirements-for-git-operations/ for more information.
fatal: Authentication failed for 'https://github.com/yourusername/yourrepository.git/'
"For developers, if you are using a password to authenticate Git operations with GitHub.com today, you must begin using a personal access token over HTTPS (recommended) or SSH key by August 13, 2021, to avoid disruption." Note that, "If you have two-factor authentication enabled for your account, you are already required to use token- or SSH-based authentication."
https://github.blog/2020-12-15-token-authentication-requirements-for-git-operations/
So it looks like we have a few more steps. We can either create a token on GitHub and use it as a password or set up SSH. SSH is quite a bit more complicated because you generate keys, add a key to an ssh-agent, and add a key to GitHub. Thankfully, once you complete these steps, you won't need to revisit them very often.
https://docs.github.com/en/authentication
Visit the links below and follow the instructions provided by GitHub.
Creating a Personal Access Token
If you'd like to create a PAT, click the link below and follow the instructions from GitHub. Note that "Personal access tokens can only be used for HTTPS Git operations."
Connecting to GitHub with SSH
https://en.wikipedia.org/wiki/Secure_Shell
https://docs.github.com/en/authentication/connecting-to-github-with-ssh
https://docs.github.com/en/authentication/troubleshooting-ssh
Cloning with SSH
Hopefully, everything went smoothly with the authentication steps. To clone a repository via SSH, click on the Code button and change HTTP to SSH. You'll notice the URL changes to something resembling a GitHub email address. Next, copy the SSH login information and revisit the command line in your Terminal App.
To download the project, type git clone
(space) and then paste (command + v) the information from GitHub, so it looks like this.
git clone git@github.com:yourusername/yourrepository.git
Hit enter
to execute the command.
Cloning into 'yourrepository'...
Now you can check the Desktop for a new folder.
cd yourrepository
To open in VS Code, enter the following.
code .
Publishing project changes to GitHub
In VS Code, open the README.md
file and add some text (e.g., Read me file).
Save the file and notice how both document margin and file change color (green and tan, respectively). Let's commit these changes back to GitHub.
Open the integrated Terminal in VS Code.
View > Terminal (or Control + `)
Add your changes: git add .
Look at the changes: git status
Commit the changes:git commit -m "Type a meaningful commit message"
1 file changed, 3 insertions(+), 1 deletion(-)
Push the changes:git push origin main
remote: Resolving deltas: 100% (1/1), completed with 1 local object.
To github.com:yourusername/yourrepository.git
00b4cf6..0aedfb5 main -> main
Getting updates from other GitHub users
You can test user changes by visiting your GitHub repo. Use the "Add file" button, then "Create new file." For the name, type "test.md". The file body says, "Edit new file" type some example text (e.g., Example). Scroll to the bottom and click "Commit new file." Now, there are changes in the repository that we don't yet have on our computer. To retrieve these changes go back to the command line and enter the following.
git pull origin main
Result:
From github.com:yourusername/yourrepository
* branch main -> FETCH_HEAD
0aedfb5..ad27b1c main -> origin/main
Updating 0aedfb5..ad27b1c
Fast-forward
test.md | 1 +
1 file changed, 1 insertion(+)
create mode 100644 test.md
You should see a new markdown file in VS Code called test.md
with the text "Example" in the file.
Way to go! You can now push and pull changes from GitHub.
To delete a project, visit Settings and scroll to the very bottom of the page. Then, click "Delete this repository" and follow the instructions.
Publishing a local project to GitHub
At the beginning of this article, I mentioned two common workflows for new projects on GitHub. So let's look at the second scenario real quick. We start with a local project and upload it to an empty existing project on GitHub.
Before we start, you can use this process to upload the backend
folder we set up in previous tutorials. However, from now on, I'll be renaming the project folder to taskhistor
—a shortened name for Task History. If your backend
folder is still on the Desktop
, you can use Terminal to rename the folder and reorganize files. Alternatively, you can create a new default GitHub project, clone the files, and revisit other steps from previous tutorials.
In this case, the command mv backend taskhistor
updates the root folder name while keeping the git information in place. Then cd
into taskhistor
and mkdir backend
. Finally, mv server.js backend
will move rather than rename because the backend
folder exists. Thus far, we've renamed the root project folder and moved server.js
to a new backend folder. You can skip the "new project files" step below because you already have a project.
In the Terminal app, navigate to the Desktop
and create some new project files.
cd Desktop
mkdir yourrepository
cd yourrepository
git init
npm init -y
touch index.html main.css app.js
Open GitHub and create a new repository with the same name (e.g., yourrepository
, taskhistor
). It's crucial that when you do this, you don't make a README file, a license, or add any other files. If you do add any files, you'll create a merge conflict. Also, by not adding any files, GitHub provides an instructions page with the exact commands you need. For example, "…or push an existing repository from the command line." Pretty nifty!
git add .
git commit -m "Add files"
git push origin main
fatal: 'origin' does not appear to be a git repository
fatal: Could not read from remote repository.
Please make sure you have the correct access rights
and the repository exists.
git branch
(should be main)
git remote -v
(no message)
"In Terminal, add the URL for the remote repository where your local repository will be pushed." In other words, navigate to your local project folder with a git repository and define the project address on GitHub where you want the code copied.
HTTPS
git remote add origin https://github.com/yourusername/yourrepository.git
SSH
git remote add origin git@github.com:yourusername/yourrepository.git
git remote -v
Result:
origin git@github.com:yourusername/yourrepository.git (fetch)
origin git@github.com:yourusername/yourrepository.git (push)
"Push the changes in your local repository to GitHub."
git push -u origin main
Result:
To github.com:yourusername/yourrepository.git
* [new branch] main -> main
Branch 'main' set up to track remote branch 'main' from 'origin'.
Success! Refresh the new repo on GitHub, add a read me and a license.
Resources
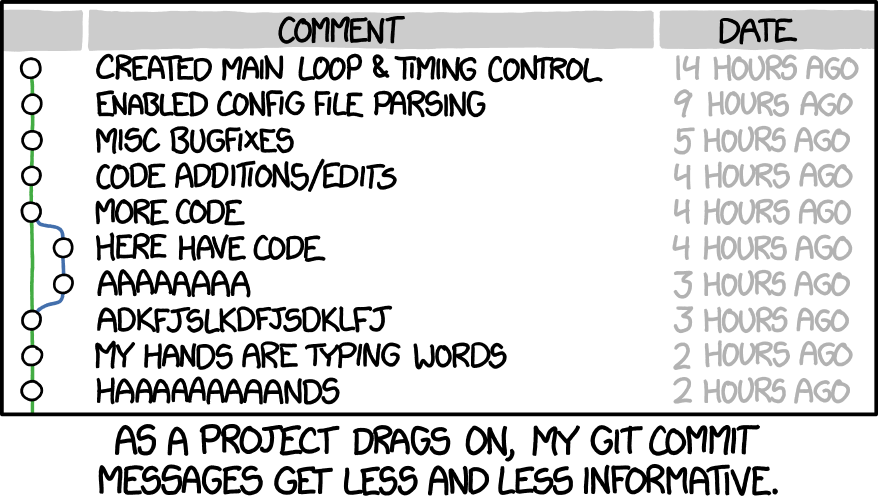
https://git-scm.com/book/en/v2/Git-Basics-Recording-Changes-to-the-Repository
Summary
This article explored how to set up and start working with GitHub using the command line. First, we created a repository, set up authentication, and cloned a repository using GitHub. Remember the main commands: git add
, git commit
, and git push
. We also explored creating a local project first and uploading it to GitHub. However, the process was a bit more elaborate. Overall, it's easier to create a project on GitHub first, then clone it locally and add your code.