Initialize Git and NPM
Git helps us track code changes, and NPM lets us leverage code resources.
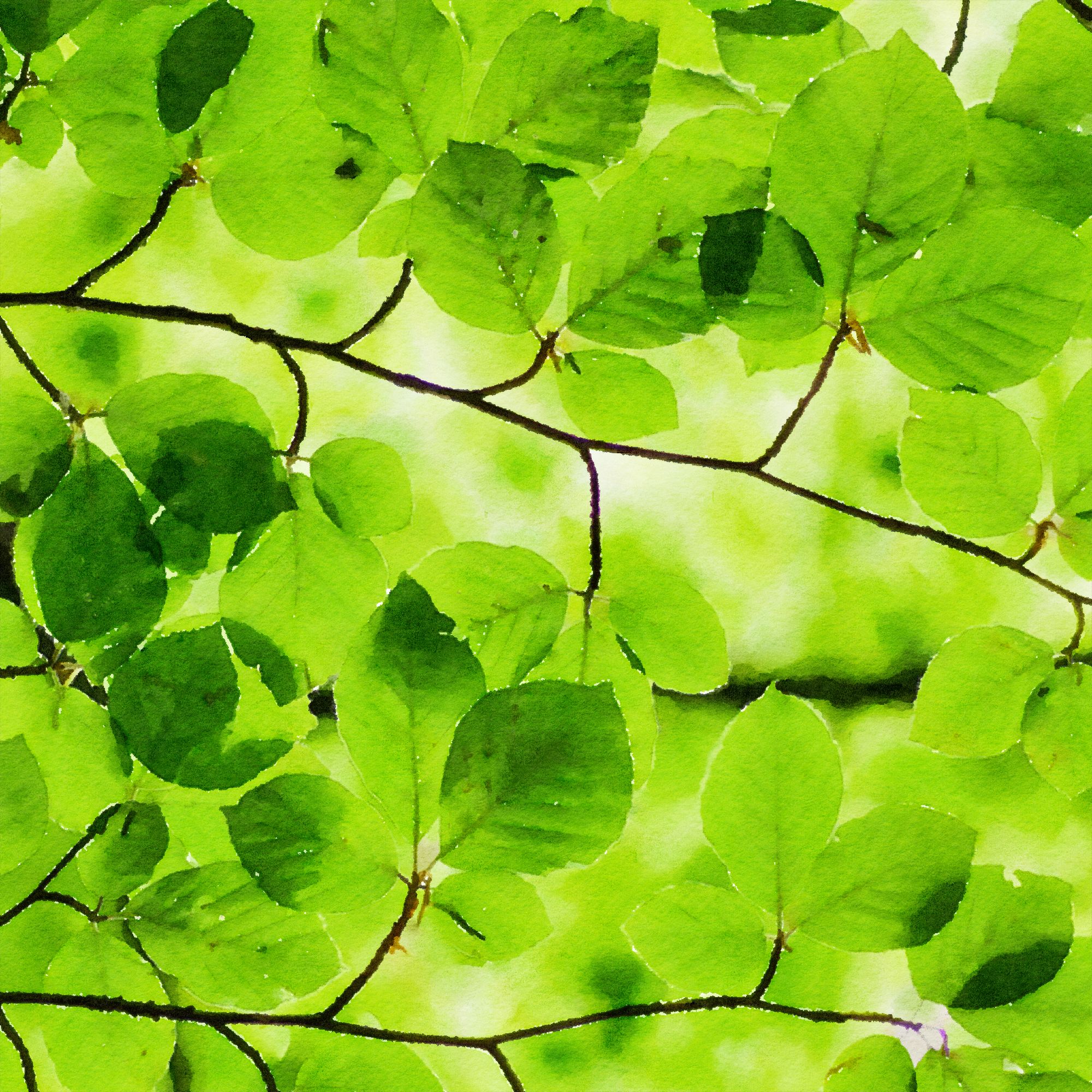
In the last tutorial, we worked with Homebrew to install Node.js and NPM. This time around, we'll look at initializing version control with Git and package management with NPM. These tools help manage complexity as projects grow.
Version control
Version control is a way of tracking changes to source code.
https://en.wikipedia.org/wiki/Version_control
Git is a tool that will track changes for us over time. Changes include folders, files, and code that is created, updated, or deleted.
https://en.wikipedia.org/wiki/Git
When you initialize a project, Git creates a master or main branch. If you make a new branch, it contains all the code of the main branch. Thus, you can experiment with new features while keeping a separate foundation of code. In addition, you and other developers can commit changes to a branch over time, which creates a history of saved work.
There are different development models for using Git and source control. One model uses a master or main branch for production, a second branch for development, and others for new features or fixes.
https://nvie.com/posts/a-successful-git-branching-model/
After completing a new feature or fixing a software bug, you merge changes back into the main or development branch.
https://www.wired.com/story/its-not-a-bug-its-a-feature/
"Software versioning is the process of assigning either unique version names or unique version numbers to unique states of computer software."
https://en.wikipedia.org/wiki/Software_versioning
Semantic versioning uses numbers with specific meaning to help manage software dependencies and track changes.
Git is essential for collaborating on teams.
https://www.atlassian.com/git/tutorials/why-git
There's a good chance you'll share the code you track locally on your computer using Git with other people. GitHub is a web interface for public or private projects. "It offers the distributed version control and source code management functionality of Git, plus its own features."
https://en.wikipedia.org/wiki/GitHub
"In software development, distributed version control... is a form of version control in which the complete codebase, including its full history, is mirrored on every developer's computer."
https://en.wikipedia.org/wiki/Distributed_version_control
Installing Git
Likely, you have Git installed on your computer.
https://git-scm.com/book/en/v2/Getting-Started-Installing-Git
For macOS, type the following into Terminal.
git --version
The result may look something like this.
git version 2.30.1 (Apple Git-130)
If you don't have Git installed already, you can use brew or an installer.
https://git-scm.com/download/mac
With Apple Git installed, I'm skipping the following command.
brew install git
Configuring Git
"The first thing you should do when you install Git is to set your user name and email address." To accomplish this step, let's look at using a private email address. Then we'll update the Git user name and email using commands shown in the guides below.
https://git-scm.com/book/en/v2/Getting-Started-First-Time-Git-Setup
https://training.github.com/downloads/github-git-cheat-sheet/
https://training.github.com/downloads/github-git-cheat-sheet.pdf
Optional email privacy
When you commit code to GitHub, your email may be publicly visible. To enable email privacy, visit settings in your GitHub account.
https://github.com/settings/emails
- User icon > Settings
- Click emails
- Activate the checkbox: Keep my email addresses private
- Copy your GitHub email
You can now paste your GitHub email address into your Git configuration (below). The format looks like this: [username]@users.noreply.github.com
Setting your commit email address
After making a commit to GitHub with your GitHub email you can try activating the setting, "Block command line pushes that expose my email."
Blocking command line pushes that expose your personal email address
Git configuration
Configure user information for all local repositories
git config --global user.name "[name]"
Sets the name you want attached to your commit transactions
git config --global user.email "[email address]"
Change the default branch
When you initialize a Git repository in the root or top-level of your project folder, it creates an invisible directory called .git with the default branch as master. Since GitHub uses the default branch of main, let's update this before initializing.
git config --global init.defaultBranch main
Verify Git settings
These configuration settings are stored in your home folder. To verify, go through the following commands in Terminal.
cd ~
ls -la
You can see a (previously hidden) folder called .gitconfig
, which contains settings.
nano .gitconfig
The opened file shows your name, email, and default branch.
[user]
name = First Last
email = username@users.noreply.github.com
[init]
defaultBranch = main
Hit ⌃X (control + x) to exit.
Configure default Git editor
We're using VS Code as our editor, so we want to configure our default editor for Git.
https://code.visualstudio.com/docs/sourcecontrol/overview#_vs-code-as-git-editor
git config --global core.editor "code —-wait"
git config --global -e
Note: the instructions provided by Microsoft to, "run git config --global -e and use VS Code as editor for configuring Git" may not work properly. If this occurs, try appending the following details into the existing .gitconfig
file.
[diff]
tool = default-difftool
[difftool "default-difftool"]
cmd = code --wait --diff $LOCAL $REMOTE
[merge]
tool = code
[mergetool "code"]
cmd = code --wait --merge $REMOTE $LOCAL $BASE $MERGED
"This leverages the --diff option you can pass to VS Code to compare two files side by side." More info.
Now VS Code will be used when Git needs you to type a message.
Initializing Git
First, we need to create a directory for our project, and then we can initialize Git in that folder. Continuing in Terminal, enter the following.
cd Desktop
mkdir backend
cd backend
git status
Result:
git status
fatal: not a git repository (or any of the parent directories): .git
The git command provides an overview of "common Git commands used in various situations."
git
git init
ls -la
You can now see a hidden file named .git
in the project directory.
git status
Result:
On branch main
No commits yet
nothing to commit (create/copy files and use "git add" to track)
If we skipped the default branch setup step, git status
would say, "On branch master" instead.
Git is now tracking our files.
Initializing NPM
"The public npm registry is a database of JavaScript packages, each comprised of software and metadata. Open source developers and developers at companies use the npm registry to contribute packages to the entire community or members of their organizations, and download packages to use in their own projects."
https://docs.npmjs.com/about-the-public-npm-registry
NPM adds the name and version to a local package.json file in your project when you install a package.
https://docs.npmjs.com/about-packages-and-modules
It's not unusual to utilize many third-party packages to build your software. However, the package.json file configuration also allows you to publish your packages. You can either go through a series of questions or use default settings to determine the values. Some of the questions or initial values may only make sense from the perspective of publishing a package. Either way, these are default settings that change over time, or you'll edit anyways.
To initialize with default settings, use the command line to enter the following.
npm init -y
or npm init --yes
In your project directory, you should see that NPM created a package.json file. Inside the file, you can look at how the information is structured using JSON. "JavaScript Object Notation (JSON) is a standard text-based format for representing structured data based on JavaScript object syntax."
https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/JSON
For now, there is nothing more to do. The important thing is that we now have a place to install JavaScript packages in the future. However, if you'd like to experiment, you can look at the other automated method of creating a package.json file.
https://docs.npmjs.com/creating-a-package-json-file
To initialize step-by-step with a questionnaire, enter the following.
npm init
The result provides some further guidance.
This utility will walk you through creating a package.json file.
It only covers the most common items, and tries to guess sensible defaults.
See `npm help init` for definitive documentation on these fields
and exactly what they do.
Use `npm install <pkg>` afterwards to install a package and
save it as a dependency in the package.json file.
At each step, you can hit return (enter) to accept default values.
Updating NPM
Even though we've installed NPM via brew, you will periodically need to update. Occasionally, NPM will prompt you with details and update instructions. Here is the primary command to know.
npm install -g npm
Uninstall NPM
We looked at installing and uninstalling Node (and npm) in the previous tutorial. To uninstall NPM directly, use the instructions on the following page.
https://docs.npmjs.com/cli/v7/using-npm/removal
Summary
The main lesson in this tutorial is to use the commands git init
and npm init -y
.
Git tracks code changes, which becomes especially relevant as our project becomes more complex.
https://git-scm.com/book/en/v2
NPM gives access to more than a million packages that can save us oodles of time and provide ongoing convenience.
https://docs.npmjs.com/about-npm
Of course, there is much more to learn about both of these tools. We'll cover more about each as it becomes relevant. Until then, you can always reference the documentation and explore if you're interested. The following article will look at a standard software design solution for full-stack web application development.