Command-line Basics
Basic command-line knowledge is a helpful tool to have in your developer kit.
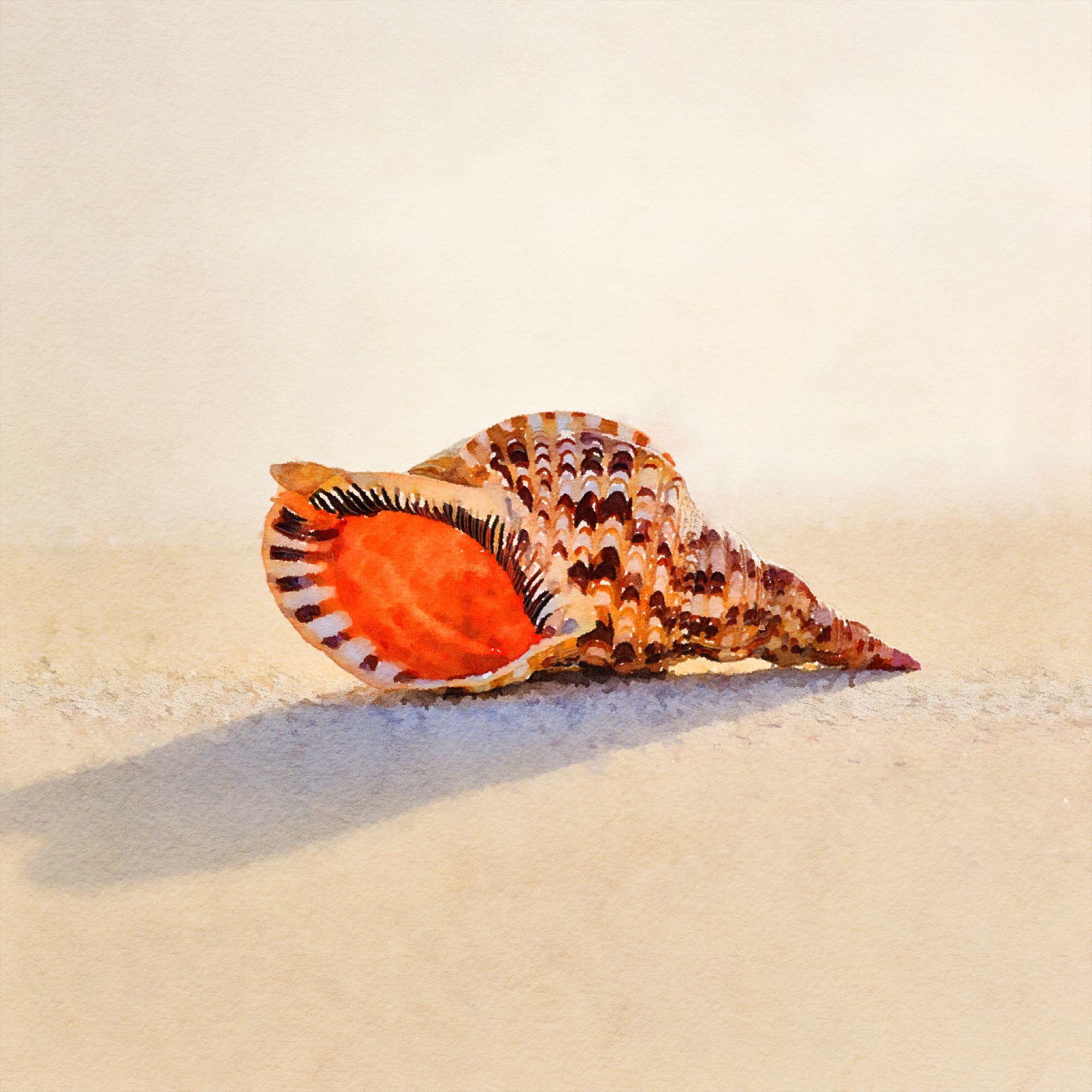
In addition to navigation and file management, I often use the command line to browse hidden files, configure cryptography keys, Git, GitHub, and occasionally interact with APIs. I also use the command line to install software and manage project packages.
This article will take you through some of the commands that are useful for web development.
Open session
On macOS, you can work with the command line by opening Terminal.
Applications > Utilities > Terminal
Apple has a command line primer here:
https://developer.apple.com/library/archive/documentation/OpenSource/Conceptual/ShellScripting/CommandLInePrimer/CommandLine.html
You can find more information in the command line manual or by visiting:
https://ss64.com/osx/
Get information
man
: help manual
e.g., man pwd
brings up more information about that command. To exit, press the q
key.
pwd
: print working directory
e.g. /Users/username
clear
: clear terminal screen
ls
: list information about a file(s)
e.g., ls -l
to list files and folders or ls -la
to see more info (including hidden files)
After listing files and folders (directories), you can navigate up or down the file system.
Navigate
cd
: change directory
e.g., cd documents
To see the documents folder contents, use ls -l
again. To navigate up a level (back to your home folder), use cd ..
or go up two levels with cd ../..
You can also navigate to your home folder using cd ~
from anywhere. Go to a specific folder by designating the full path like:cd documents/developer
.
You can also type cd
, space, and drag a folder from the Finder into the Terminal window. Adding the full folder path like this can be a big time saver.
If a folder name contains spaces, use single quotes around the words like cd 'web dev’
To autocomplete, start typing cd Desk
, then press tab to complete Desktop
and enter. The path to be completed should be a subfolder.
You can cycle through previous commands by pressing the up arrow (on your keyboard).
Create folders and files
mkdir
: create a new directory (folder)
e.g., mkdir project
makes a project folder and then cd project
moves you into that directory.
touch
: create new files, change file timestamps
e.g., touch index.html main.css app.js
Creates three files in the project folder (created above).
open
: open a file/folder/URL/Application
e.g., open project
Opens the example project folder (created above). You can open a file like index.html with open index.html
. In this case, the file is empty but still opens in your default browser.
Text editing
nano
: simple text editor
e.g. nano index.html
then paste the following boilerplate code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<p>Hello world!</p>
</body>
</html>
To exit press ⌃X
(control + x)
Save modified buffer (ANSWERING "No" WILL DESTROY CHANGES)?
Yes.
File Name to Write: index.html
Press enter.
Now try the command open index.html
again. Result: Hello world!
shows on a web page in your browser.
Rename or move
mv
: move or rename files or directories
e.g., mv project app
If a folder called app doesn’t exist, change its name to project. If you make a new directory on the Desktop called app and try the same command, you’ll move the project folder inside the app folder instead: ~/Desktop/app/project
. To undo this, you could move again with: mv ~/Desktop/app/project ~/Desktop
Alternately you can use a single period to indicate a path from your current directory: mv ./app/project
Copy files
cp
: copy one or more files to another location
e.g., cp project/index.html app
Copies index.html to the app folder. If the index.html file already exists in the app folder, it will be replaced (i.e., deleted).
For more information about recursive copying, see:
https://ss64.com/osx/cp.html
Delete files and folders
Be careful with this command, or you can end up deleting your root directory (entire computer) or your home folder (entire user directory). Always specify what folder you’re removing and be very aware of your current directory. For more information, see https://ss64.com/osx/rm.html
rm
: remove files. Delete files and folders.
e.g., rm ~/Desktop/project/main.css ..
The main.css file is deleted.
rm -rf app
The app folder disappears, which contained a copy of index.html.
Flag -r
removes the entire file directory (recursive)
Flag -f
deletes without confirmation
Delete folders
rmdir
: remove a directory (delete folders)
e.g., rmdir project
Result: rmdir: project: Directory not empty.
To delete the remaining files, you could do:rm ./project/index.html ./project/app.js
With the directory now empty, try the rmdir project again. The folder is deleted.
Authentication
Occasionally, you may need the su
or sudo
command for installing software or changing permissions.
https://en.wikipedia.org/wiki/Sudo
su
: substitute user identity.
“If su is executed by root, no password is requested.”
sudo
: execute a command as another user
https://ss64.com/osx/sudo.html
Change permissions or owner
It’s good to be aware of these commands—you might need them occasionally to reset or troubleshoot permissions locally or on a server.
chmod
: change access permissions, change mode
https://ss64.com/osx/chmod.html
(Note: the permissions calculator on this page is super helpful!)
https://en.wikipedia.org/wiki/Chmod
chown
: change file owner and group
https://ss64.com/osx/chown.html
https://en.wikipedia.org/wiki/Chown
Servers
It’s good to be aware of these commands—you might need them occasionally to troubleshoot access to an API or configure certificates.
https://en.wikipedia.org/wiki/Internet_protocol_suite
https://en.wikipedia.org/wiki/Port_(computer_networking)
https://en.wikipedia.org/wiki/List_of_TCP_and_UDP_port_numbers
curl
: transfer data from or to a server
https://ss64.com/osx/curl.html
ssh
: OpenSSH SSH client
https://ss64.com/bash/ssh.html
ftp
: internet file transfer program
https://ss64.com/bash/ftp.html
ping
: test a network connection
https://ss64.com/osx/ping.html
openssl
: OpenSSL command line
https://ss64.com/osx/openssl.html
https://en.wikipedia.org/wiki/OpenSSL
https://en.wikipedia.org/wiki/Comparison_of_cryptography_libraries
Close session
exit
or logout
: exit a login shell and save history
There’s more command line yet to learn including: installing software, packages, and file tracking with Git and GitHub.
In the following article, we'll install VS Code.
https://www.gamesoflight.com/install-vs-code/